Customize Menu Items in Drupal User Profile
We were recently asked by a client to edit the user profile view page on their site. This client needed us to move the link to the user’s contact form out of the tab area at the top of the profile and replace it with a link that appears further down in the content of the user’s profile. While this is not something you can do through the admin interface in Drupal 7, it is easy to do with just a few lines of code in a custom module, which I will show you how to do here.
Prior to adding our custom code, the link to the contact form appears as a tab.
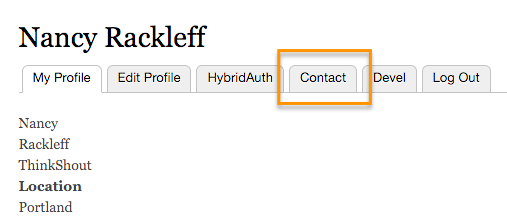
The “Contact” menu item starts out as a tab because the Drupal contact module originally creates the menu item and assigns it the type MENU_LOCAL_TASK. (See Menu item types for a list of the possible menu types and their uses in Drupal.) In order for us to change the type, we can use Drupal’s hook_menu_alter() function to change the item to the MENU_CALLBACK type, which will remove it from the display, but keep it available as a valid path.
/**
* Implements hook_menu_alter().
*/
function mymodule_menu_alter(&$items) {
// Remove the 'contact' tab.
$items['user/%user/contact']['type'] = MENU_CALLBACK;
}
Now it is no longer a tab, but we still need make use of Drupal’s hook_user_view_alter() to insert it into the content of the profile before it is rendered on the page.
/**
* Implements hook_user_view_alter().
*/
function mymodule_user_view_alter(&$build) {
// Check to see if this user has allowed others to contact him/her.
if ($build['#account']->data['contact']) {
// Create the text for the link using the account info to get the user’s first name.
$link_text = $build['#account']->field_first_name['und'][0]['safe_value'] ? "email "
. $build['#account']->field_first_name['und'][0]['safe_value'] : "email";
// Use the l() function to create the link.
$contact_link = l($link_text,'user/' . $build['#account']->uid . '/contact');
// Insert it into the $build array.
$build['contact_link'][0]['#markup'] = "
";
// Insert into the user details that group we created in the display mode in admin interface.
$build['#group_children']['contact_link'] = 'group_user_details';
}
}
After the custom code and a quick cache clear, the tab is gone and there is a link to the form within the body of the profile.
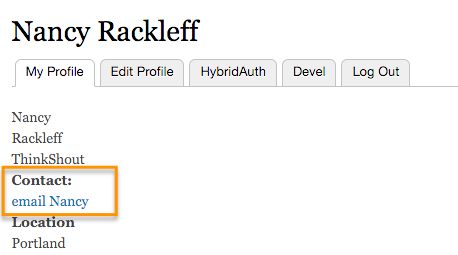
I won’t go into creating a custom module; that’s a bit beyond the scope of this post, but there is a tutorial for creating a custom module on drupal.org.
Shout out to Greg Boggs for his assistance!